3 minute read
DRAFT
count-controlled loop debug efficient iteration nested loop repeat block
Now try this!
Work in pairs. Write an algorithm to direct the robot in square B5 to the star in square E5, following the white road. Use commands like these to write your instructions: Forward 3 (to move forward 3 squares), Turn right. When moving, you should not count the square the robot is currently on. For example, the command ‘Forward 3’ will move the robot to square B2.
1.3 Count-controlled loops
Count-controlled loops
Forever loops repeat instructions forever. But it is more common to use a loop that repeats an exact number of times. This is called a count-controlled loop
Once a count control has finished its number of repetitions, it can leave the loop and continue with the rest of the instructions. If there are no more instructions after the count-controlled loop, the algorithm will end. Look at the algorithm below for crossing the road. We repeat the
Iteration is another name for a loop. A count-controlled loop is one example of an iteration.
Iteration makes algorithms and programs more efficient . An efficient program will run faster and use less power and computer memory. The best way to create an efficient algorithm is to get rid of lines of code that repeat and use iteration instead.
Iteration repeats the instruction or code, so you don’t have to write it lots of times.
Think about the robot puzzle in the Getting started activity.
We could write the instructions as:
But it is easier to use a count-controlled loop, like this:
The number next to the word REPEAT tells the algorithm how many times to repeat the instructions.
In the second algorithm the robot turns right three times. The last turn is unnecessary. So, which algorithm is more efficient?
The extra turn in the second algorithm doesn’t change the outcome. The algorithm still moves the robot to the star. An algorithm should be short and concise. The first algorithm has five instructions. The second algorithm has only three instructions, so it is still more efficient.
This loop could also be shown like this:
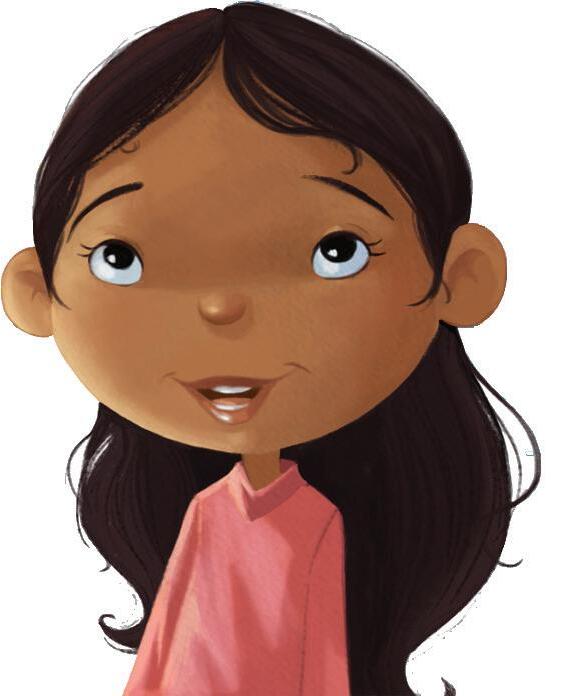
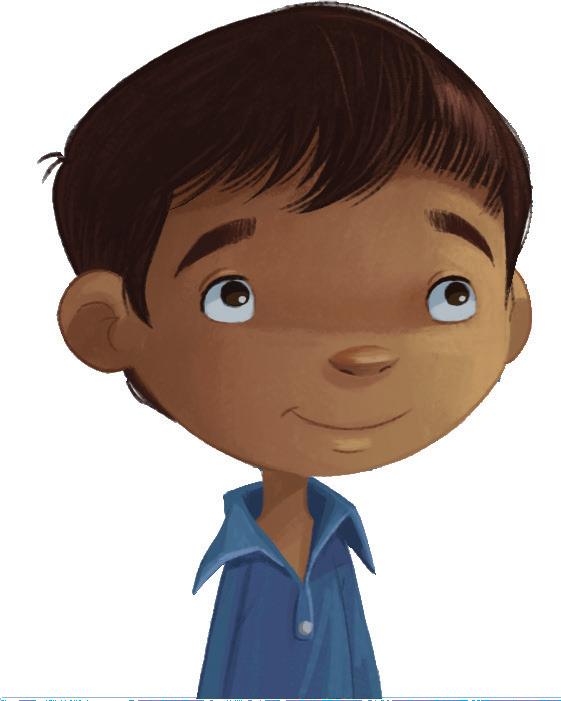
Why does this diagram have a stop instruction at the end but some of the other diagrams we’ve looked at don’t?
An indefinite loop keeps repeating forever so will not stop. The count-controlled loop repeats a number of times and then the loop stops.
Follow this iteration algorithm. Use your finger to trace your way through the maze.
Using iteration makes programming much faster because programmers don’t need to type the same line of code lots of times.
Remember, debug means to find and correct errors in code.
Programmers can also debug their code more easily. This is because programmers only have to correct an error once, instead of lots of times.
Questions
Work in pairs to answer these questions.
1 What is the difference between an indefinite loop and a count-controlled loop?
2 Why do programmers use loops?
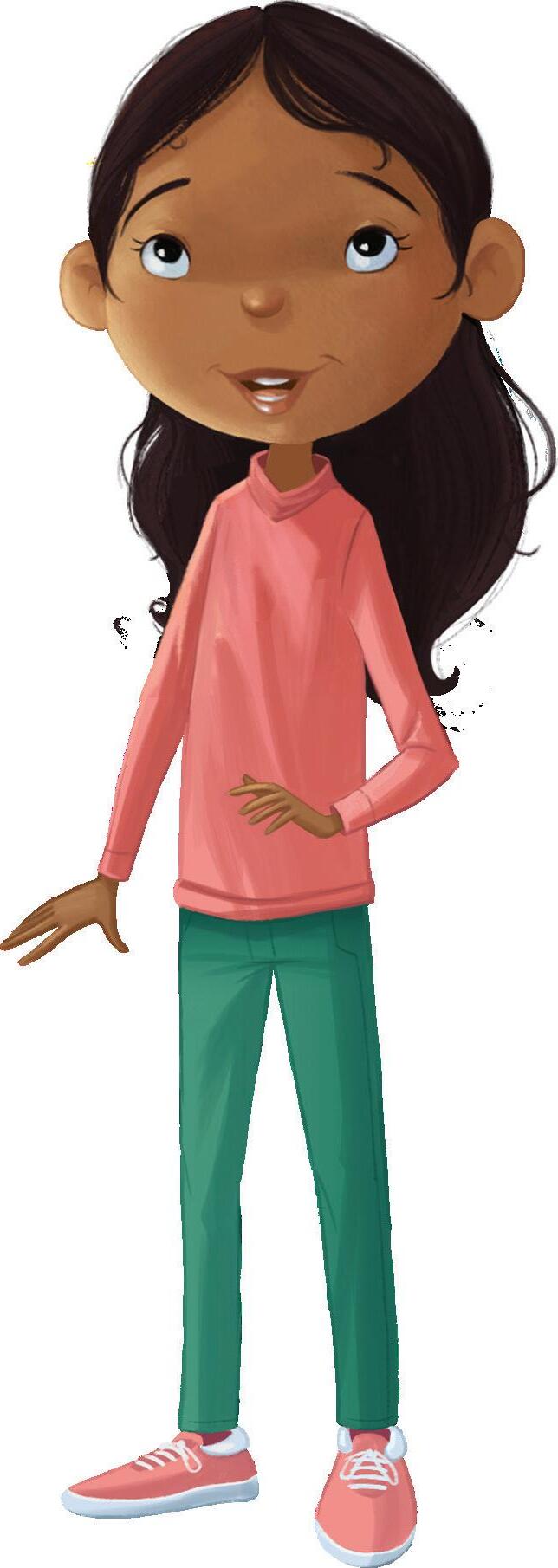
1.3 Count-controlled loops
Correcting count-controlled loops
When trying to debug algorithms and programs, you should follow the iteration to find out where the program went wrong. Once you find the error you can correct it.
Unplugged activity 2
You will need: paper and a pen
Look at this simple maze. The robot should follow the path in a square until it gets back to where it started. Follow the algorithm that is written below the maze. Debug the algorithm.
Nested loops
Sometimes a loop can contain smaller loops inside it. When a loop is inside another loop it is known as a nested loop nested loop
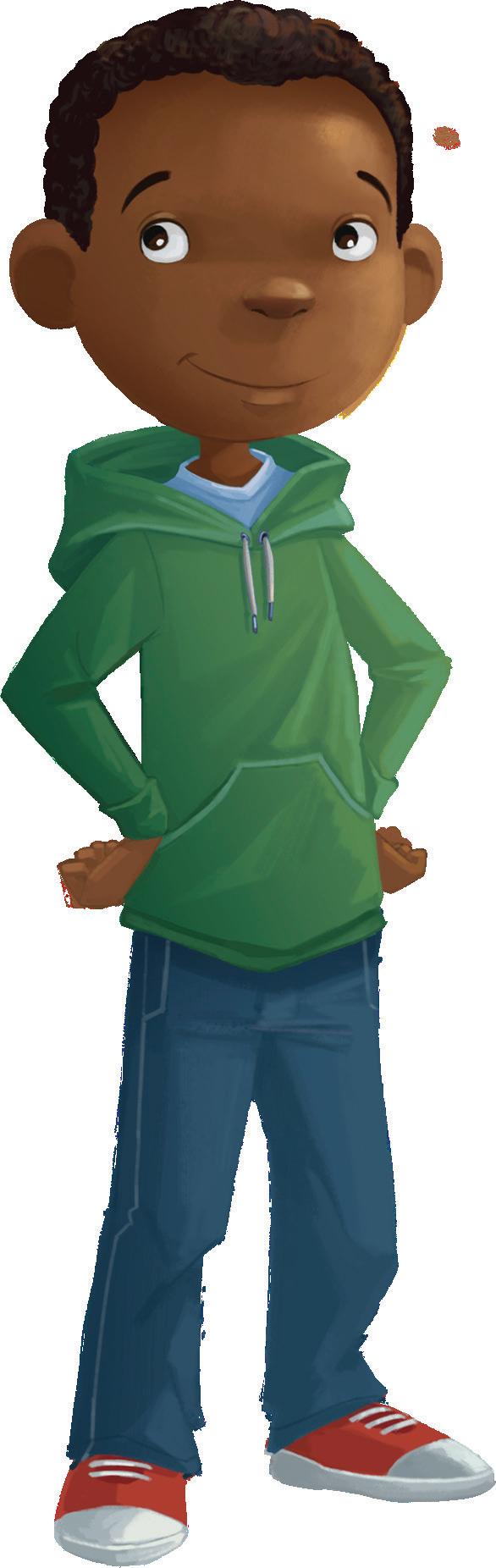
Robots are now used in some restaurants to take orders and serve food to the customers. The programming for robot waiters is very complicated, but a simple algorithm for them is shown below. This algorithm shows how a robot waiter takes orders for food and brings the food to the customer’s table.
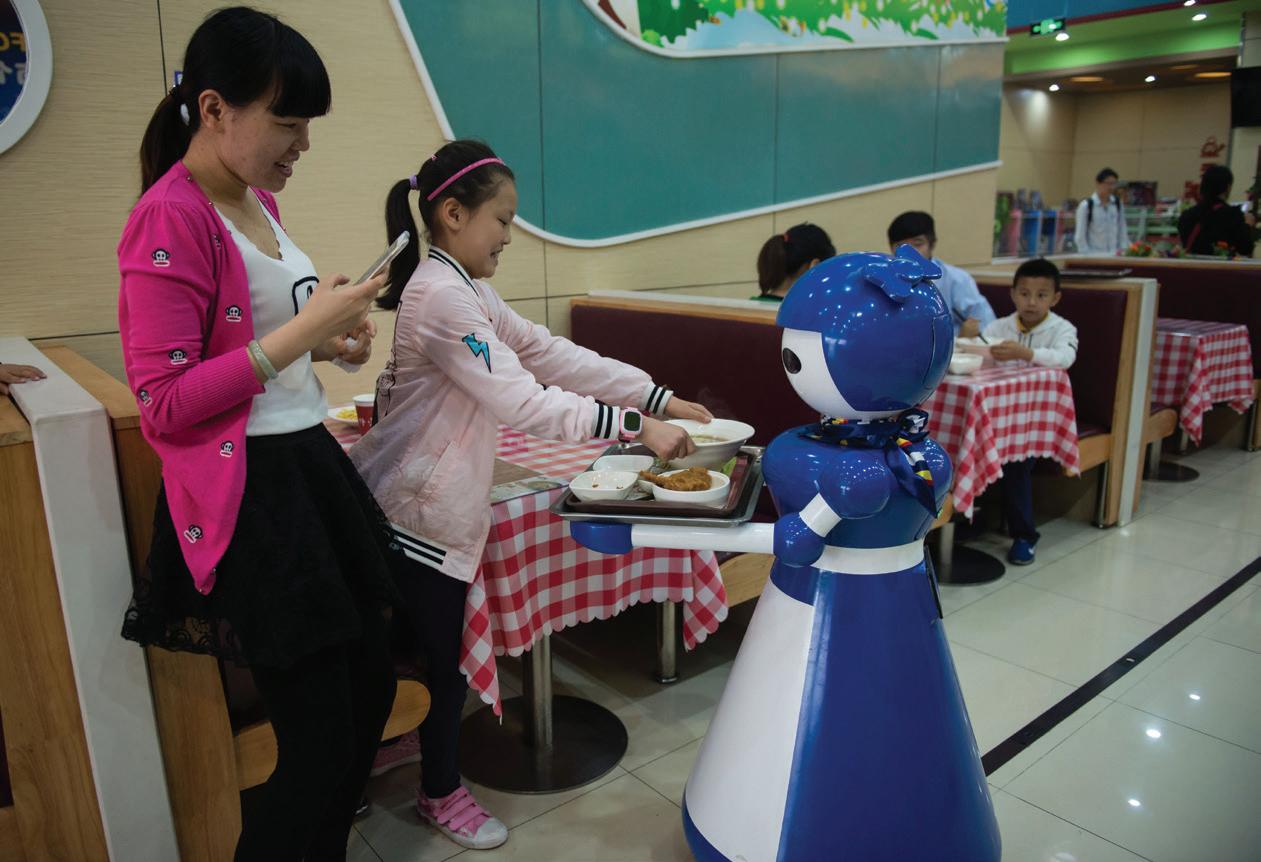
1 REPEAT FOREVER.
2 Go to table.
3 Welcome customers.
4 Count how many people are at the table (save this number as ‘N’).
5 REPEAT ‘N’ times.
6 Ask 1 person for their order.
7 Send this order to the kitchen.
8 Move onto the next person.
9 When food is ready and put on tray, pick up tray.
10 Bring food to table.
Did you notice that the code is indented? The position of the text is very important when you are using nested loops as it shows where the loops start and finish.
1.3 Count-controlled loops
The robot waiter repeats the process to take the order from each person using a count-controlled loop. The count-controlled loop is inside a bigger indefinite loop that repeats until the waiter is switched off. The count-controlled loop is a nested loop.
Did you know?
Digital clocks and timers use nested count-controlled loops and indefinite loops. The seconds are repeated 60 times, the minutes are repeated 60 times and the hours in the day are repeated 24 times. These are all count-controlled loops. The whole sequence is repeated in an indefinite loop.
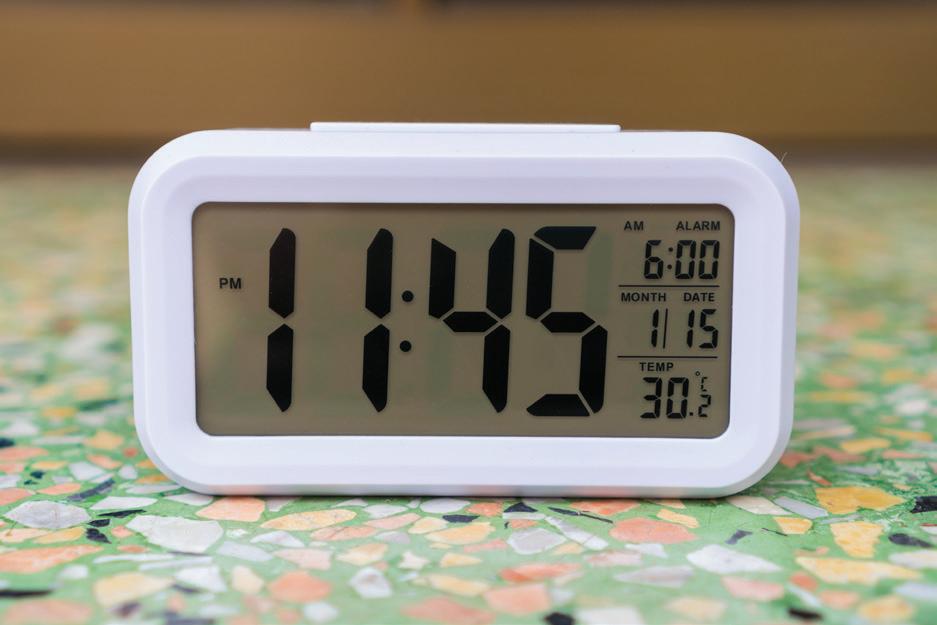
Unplugged activity 3
Learn a new dance together as a class.
1 Read the instructions in the algorithm.
2 Follow the instructions to do the dance together.
3 Say the underlined words when you do each action. (This will help you follow the instructions.)
1 REPEAT 3.
2 Jump once on the spot.
3 REPEAT 4.
4 Take a step to the
5 Take a step to the
6 Clap your hands.
7 Wave your hands above your head.
8 Take a bow.